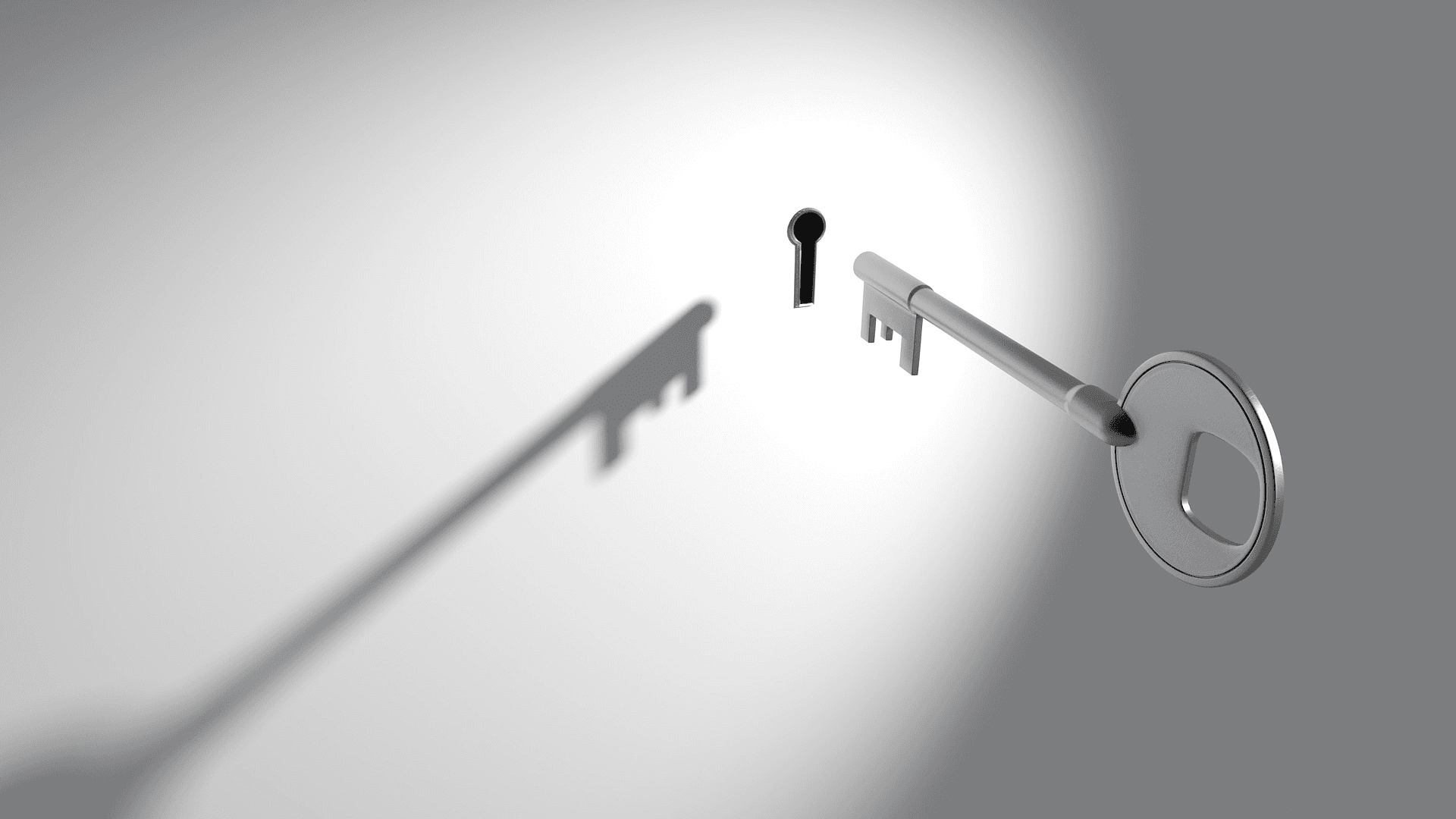
AWS Secrets + Serverless
Date published: 17-Nov-2022
1 min read / 103 words
Author: R.G.
AWS
Secrets Manager
TypeScript
Instead of storing secrets in your .env
files and/or environment variables, it is safer to programatically fetch secrets via a store like AWS Secrets Manager. This is because if a hacker can obtain Remote Code Execution (RCE) reading your environment variables is trivial at that point.
If you are using TypeScript it is quite simple to fetch your secrets:
import { SecretsManagerClient, GetSecretValueCommand } from '@aws-sdk/client-secrets-manager';const client = new SecretsManagerClient({region: 'us-east-1',});export function getOverrides() {return client.send(new GetSecretValueCommand({SecretId: 'my-secret-name',})).then(({ SecretString }) => {if (SecretString) {return JSON.parse(SecretString);}throw new Error('Could not find secret string');}).then(({ privateKey, webhookSecret }) => ({privateKey: privateKey.replace(/\\n/gm, '\n'),secret: webhookSecret,})).catch((err: Error) => {throw err;});}
This code is run in the context of a Lambda function, and then passed to one of our libraries. All we need to pass is the region our secret is stored in, parse it, format if necessary and we are off to the races!